In this article I’m going to write a quick introduction to intel x86 assembly language. We are going to create a program, that is going to print a sentence in the screen.
Before starting programming, we need to know a couple of things, what is a system call, what is the instruction 0x80 and what are assembly registers.
- A system call, is a the programmatic way in which a computer program request a service from the kernel of the operating system it is executed on.
- Int 0x80 is the assembly language instruction that is used to invoke system calls in Linux on x86 (i.e., Intel-compatible) processors.
- Also we need to know what are assembly registers EAX, EBX, ECX, etc. A register store data elements for processing without having to access the memory, and all these are General Purpose registers.
In the image below, you can see the content of the file “/usr/include/i386-linux-gnu/asm/unistd_32.h” that contains all the linux 32 bits system calls.
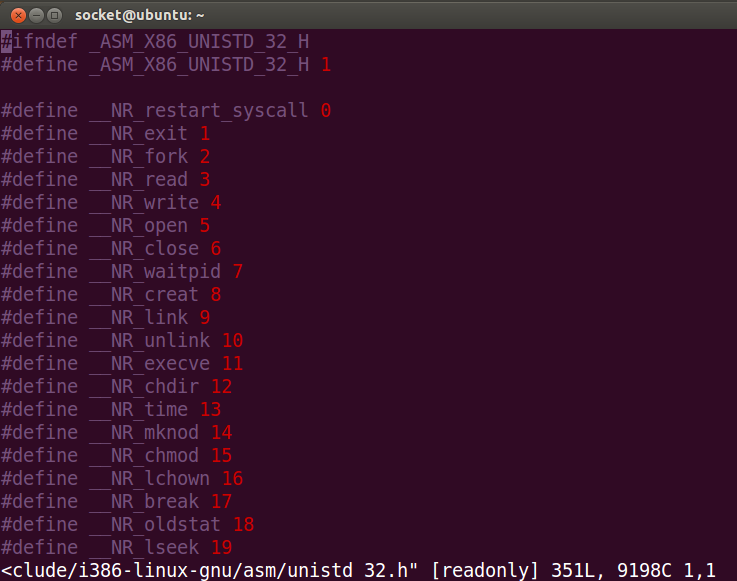
We can see that the system call write is the number 4. To see in more detail what is this system call and how to use it, we can execute the command “man 2 write”.
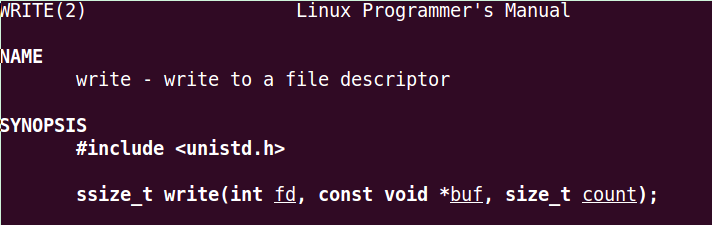
This sytem call, needs 3 arguments. To set them to the value that we need, we just have to use the assembly registers:
EAX – 1st argument
EBX – 2nd argument
ECX – 3rd argument
EDX – 4th argument
ESI – 5th argument
EDI – 6th argument
So, lets write “Hello World!” using write syscall:
- EAX has to be 4, because write is the syscall number 4.
- EBX, needs to be 1, because we want to write to file descriptor 1, that is stdout.
- ECX needs to contain the message, in this case the variable message.
- EDX, needs to contain the length of message, that is 12.
The last thing we need to do, is to use 0x80 to invoke the system call.
So this is the final code:
; write syscall
mov eax, 0x4
mov ebx, 0x1
mov ecx, message
mov edx, 12
int 0x80
And this will be the full program:
; HelloWorld.asm
; Author: Xavi Beltran
global _start
section .text
_start:
; write syscall
mov eax, 0x4
mov ebx, 0x1
mov ecx, message
mov edx, mlen
int 0x80
; exit syscall
mov eax, 0x1
mov ebx, 0x0
int 0x80
section .data
message: db "Hello World!"
mlen equ $-message
To compile it we must run:
nasm -f elf32 -o HelloWorld.asm -o HelloWorld.o HelloWorld.asm
ld -o HelloWorld HelloWorld.o
And here you can see the execution of the program:
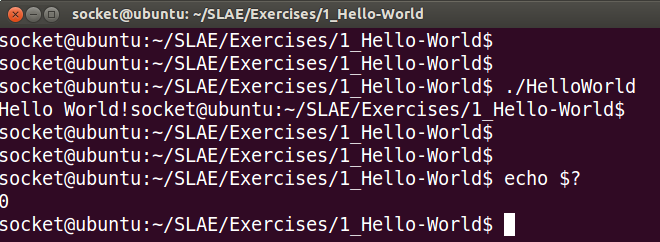
That’s all for the first introduction to assembly language!